Getting Started
Introduction
The Open Banking API (Application Programming Interface) is a web service offered by Brüc + Bond to TPPs (Third Party Providers) to simplify the integration process with Brüc + Bond's systems. This API allows TPPs to easily interact with the Brüc + Bond Platform. Via the API, TPPs can retrieve information about accounts, balances and transactions, as well as create new transactions.
Definitions
Term | Definition |
---|---|
AISP | Account Information Services provide consolidated information on Client payment accounts held at one or more PSP(s). |
ASPSP | Account Servicing Payment Service Providers provide and maintain a payment account for a Client. |
CBPII | Card Based Payment Instrument Issuers are PSPs that issue card-based payment instruments. |
Client | A bank account holder. Brüc + Bond Clients can only ever be legal entities. |
PISP | Payment Initiation Services Providers facilitate the initiation of payment order(s) to an account held at another PSP on the request of a Client. |
PSP | A Payment Services Provider is an entity which carries out regulated payment services, including AISPs, PISPs, CBPIIs and ASPSPs. |
TPP | Third Party Providers are legal entities or natural persons that use APIs to access Client accounts in order to provide account information services and/or to initiate payments. |
PSU | A Payment Service User is a person authorised to access and/or perform actions on a Client account held with Brüc + Bond. |
SCA | Strong Customer Authentication is a requirement of the PSD 2.0 framework to ensure that payments are performed with multi-factor authentication. |
Become a TPP
TPP Registration
curl -X POST "https://api.brucbond.com/api/v1/third-party-provider"
-H "Content-Type: application/json"
-d '{
"name": "TPP name",
"certificate": "eIDAS certificate pem",
"email": "tpp.name@email.com",
"phoneNumber": "your.tpp.phone.number"
}'
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true,
"result": "Success. Instructions were sent to your email address."
}
The process of TPP registration is initiated via a request made to the Brüc + Bond API. Following the request, the TPP certificate is validated and access is granted on successful validation.
HTTP Request
POST https://api.brucbond.com/api/v1/third-party-provider
Body Parameters
Parameter | Type | Description |
---|---|---|
Name | String | The company name of the registering TPP. |
Certificate | String | The eIDAS certificate confirming the TPP's license as a service provider. |
String | Email address for the contact person at the TPP. | |
PhoneNumber | String | Phone number for the contact person at the TPP. |
Application Registration
curl -X POST "https://api.brucbond.com/api/v1/application"
-H "Content-Type: application/json"
-H "tpp-key: <TPP_KEY>"
-H "tpp-secret: <TPP_SECRET>"
-d '{
"name": "Your application name",
"redirectUri": "https://your-application-domain.com"
}'
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true,
"result": "Success. Application key and Application secret were sent to your email address."
}
The TPP application may refer to a website, mobile application, and so on. The client authorizes access for the TPP's application to use the API resources. This authorization is acquired via the Access Authorization flow.
HTTP Request
POST https://api.brucbond.com/api/v1/application
Body Parameters
Parameter | Type | Description |
---|---|---|
Name | String | Name (label) of the application. |
RedirectUri | String | URI to which user is redirected after authorization. |
Authentication
The Brüc + Bond API is JSON based. In order to make an authenticated call to the API, you must include your access token with the call. OAuth2 uses a BEARER token that is passed along in an Authorization header.
After successfully registering as TPP, you will be given a set of 2 unique keys:
APPLICATION_KEY
APPLICATION_SECRET
These two keys are subsequently used to obtain access tokens for making API calls.
Authentication Flow
The Authentication endpoints are as follows:
- Authorization:
https://account.brucbond.com/#/login/oauth/v2/authorize
- Token Request:
https://api.brucbond.com/token
Authorization Code
The Authorization Code flow is a redirection-based flow, which means the application must be able to redirect the application user and receive authorization codes via a web browser
Phase 1: Authorization Phase
open https://account.brucbond.com/#/login/oauth/v2/authorize?app-key=<APPLICATION_KEY>
Make sure to replace
<APPLICATION_KEY>
with your details.
An authorization code is the key used to retrieve the access token.
In order to acquire an authorization code, you need to redirect the user's
browser to the authorization endpoint.
https://account.brucbond.com/#/login/oauth/v2/authorize?app-key=<APPLICATION_KEY>
https://account.brucbond.com/#/login/oauth/v2/authorize
: indicates the authorization endpoint.app-key=<APPLICATION_KEY>
: the application's{app-key}
provided when registering your application.
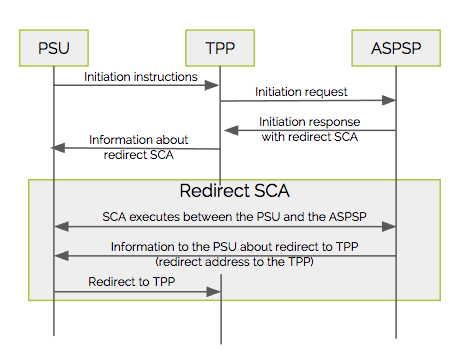
Phase 2: User Authorization
Once directed to the above link, the user will be asked to log in to their Brüc + Bond account. They will then be asked to define the level of access (scope) for his account.
Phase 3: Authorization Code response
After the user successfully authorizes the application, they will be redirected back to
the {redirectUri}
(provided during application registration) with the authorization code as a query parameter named app-authentication-code
.
e.g. https://my.tppawesomeapp.com/auth/callback?app-authentication-code=123c6348c57ccv920
Phase 4: Requesting an Access Token
curl -X POST "https://api.brucbond.com/api/token"
-H "Content-type: application/x-www-form-urlencoded"
-d 'grant_type=authorization_code&code=<AUTHENTICATION_CODE>&client_id=<APPLICATION_KEY>&client_secret=<APPLICATION_SECRET>'
The above command returns JSON structured like this:
{
"access_token": "wyloYWu4bYBq3bxp-n5HuRM5QsgDGv8cUo5g9vmlqd_-RSjKySPNfEx4enhx_GkDqKxr34zA8uTwUWZJQbaJb6gvCoUHTeps5cIfySOMqoxkuxxp664w_BvlO-oZn-fkMElxKdL77Ts8jIq0C2eRRcD0APJc_tfx2GnN721sO1K33grJYhfKo-472-mQ4dwEykCogwWvkovAWmzDAt4DaXAeg2dKwHrgSah6T-qwvThR9hUWwzV4Zq5mXxQJCDwhL5iwgBUQXocMbe4vx_WAom30LIZcxdNpfBlUpticWHGJvnmwbFMEyNmPGegW516EP2daPK2E3K5nYtXjPBmM9jxiuukVITR9Ax7WhkscUFK3l200kJSfm9SH_qgQTbK92p61tXp2O3R2D0maGHuBlE_B1kBN5qETWAOIWPOLGKI0QTR7pPdyhyaNzyRdsUwQQXsvBPZDmVDPabCpJVqnKFiBxr_HyQjvuh0IG970f8f9OoBPBOGP83SWu7zKIpjttIxB96LIJhiS8js13weX-PCPQGUGFq8__9hEdhTD_8ri6Ias1hRil9jG3dmA0Xn8RQqfKqsRWVPZgIuFRt0fVf7282Za0kwzZ92VVAU3x9AIP7jHgXpJAAJM4Rx8JW9cGh0iN_uGWwCunYyJ6VVwbL9Qnz3FgNTW4KRg8klsHbM-_tJdB3sJF0PzeNrkyy3Q_lSCPJwETkuBM1rB0vUSkA",
"token_type": "bearer",
"expires_in": 7775999,
"refresh_token": "dBgPgajBTz55ERgOoobwCZk_8IjsWm9sIUy35zAsYvVBiDaxhQ_hXeFyFJl0DAHo0IkrJMJTqnapUiizlDiCTmKgE1LxgyN7U5LsEendDqJIAHLHZYhrkGdMXcCfUfU-cbvhIEd1t6W7ePBdGGYENcYgRZNNOM0_jp7SshaTh03agpvFh2fIfMfr32EzJVamLetIxWQRk7aQLM7XuJHrA8IgLItCzIxJibe19vfJOpltqS0Zo4fhALrcDvyIH2PwHdZRHlHHurTPtKtvNZe-ZJkvIak8Nnf6snkVmcVkkIkfjac6JjFRn7QOqzl64CPRzEGYZaXDIc8D6V6TAKefa_ZQHwG-bSr7NmPKp9Xh2ruHyfjyFUp_m5GMXaFBXKnUoxqvsjCTvW_zNPMLig0OI_vMO0jRC2UMT4syEat3IDGQwvNi2D90ABYwT3Qc0ZX8wzz3BLyvvuKIz63Q47HsG1OyaArjrX6pHLkYiroCuyNz8tBMpZRfymQsGjurDTd8CCdTdov6FvoiD-dEzoNTOE1pzJouRRgR06hNxYKae2xSMOCka70ozVGekB6aBjop2Cis3BqCeuPfJNc5QGNc8LvjuKXfrR8x6Js_Avgb9BnmjtWvn20b0pM5_eienn6XFucNo1pamxzEtXZkR7UagfA_YWGgLogKST9GPyhkcGnLrIURR_1jikZ2mNs9KhGBBuCXTt8FQRoOnCEyBwGR5A",
"userName": "Mike.Wazowski@gmail.com",
"fullName": "Mike Wazowski",
"lastLogin": "04.05.2020 10:25",
"id": "60b70f08-1ab3-49ff-a619-d41954aa4cdb",
"role": "Client",
"companyRolesJson": "{\"12\":[\"Company\"]}",
"permissionsJson": "[]",
"currentCompanyId": 1212,
"client_id": "bc893656-add5-4552-9079-b0703e4c1b51",
".expires": "Sun, 02 Aug 2020 08:27:45 GMT",
".issued": "Mon, 04 May 2020 08:27:45 GMT"
}
The access token is a unique key used to make requests to the API.
In order to get an access token, the application must make a POST request to
https://api.brucbond.com/token
with the grant_type
,
code
, client_id
and client_secret
as parameters.
grant_type=authorization_code
: specifies that your application is requesting an authorization code.code=<AUTHENTICATION_CODE>
must match the authorization code returned by the authorization endpoint in Phase 3.client_id=<APPLICATION_KEY>
: the application's ID provided when registering your application.client_secret=<APPLICATION_SECRET>
: the application's secret provided when registering your application.
Consent
TPP can check if consent is present and it’s expiration date (via GET
request) and send us a notification that user has granted the consent (via PUT
request). PUT request will also contain expiration date in it’s response.
Get Consent
curl -X GET "https://api.brucbond.com/api/v1/consent"
-H "Content-Type: application/json"
-H "Authorization: Bearer <ACCESS_TOKEN>"
-H "app-key: <APPLICATION_KEY>"
-H "app-secret: <APPLICATION_SECRET>"
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true,
"result":
{
"expirationDate": "2019-12-03T17:06:34.707"
}
}
This endpoint checks if the client gave his consent for the TPP to access his account data. The endpoint returns the content of an account information consent object.
HTTP Request
GET https://api.brucbond.com/api/v1/consent
Header Parameters
Parameter | Description |
---|---|
app-key | The application's client ID provided when registering your application. |
app-secret | The application's client secret provided when registering your application. |
Authorization | Authorization token granted during the authorization process. |
Create Consent
curl -X PUT "https://api.brucbond.com/api/v1/consent"
-H "Content-Type: application/json"
-H "Authorization: Bearer <ACCESS_TOKEN>"
-H "app-key: <APPLICATION_KEY>"
-H "app-secret: <APPLICATION_SECRET>"
-H "Content-Length: 0"
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true,
"result":
{
"expirationDate": "2019-12-03T17:12:56.30"
}
}
This endpoint creates a resource for the TPP, as per the client's consent, to access the client's account data which is specified in the request.
HTTP Request
PUT https://api.brucbond.com/api/v1/consent
Header Parameters
Parameter | Description |
---|---|
app-key | The application's client ID provided when registering your application. |
app-secret | The application's client secret provided when registering your application. |
Authorization | Authorization token granted during the authorization process. |
Sandbox
The sandbox is the place where you can get to know our Brüc + Bond API. It provides the same API as our production environment but with mock data.
Using the API in the sandbox
To use the Brüc + Bond API in the sandbox, simply replace the https://api.brucbond.com/
with
https://sandbox.brucbond.com/
Test credentials
Parameter | Value | Description |
---|---|---|
userName | user.sandbox.001@gmail.com |
The PSU username to be provided on login to PSU account. |
password | Sitepassword001 |
The PSU password to be provided on login to PSU account. |
pin | 123456 |
The PSU 2FA pin code to be provided on login to PSU account. |
OTP | 1111 |
The PSU One-Time-Password to be provided in Payment Confirmation. |
Account Information (AIS)
The Account & Transaction API gives read-only access to the client's account information and transaction data. Access is granted through an explicit consent, which must be provided to the AISP by the client.
Get Accounts
curl -X GET "https://api.brucbond.com/api/v1/accounts/all"
-H "Content-Type: application/json"
-H "Authorization: Bearer <ACCESS_TOKEN>"
-H "app-key: <APPLICATION_KEY>"
-H "app-secret: <APPLICATION_SECRET>"
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true,
"result":
[
{
"bankAccountId": 45,
"bankName": "Commerzbank",
"balanceAmount": 100155.00,
"pendingAmount": 32654.15,
"minBalance": 500,
"currency": "EUR",
"iban": "DE89 3704 0044 0532 0130 00"
},
{
"bankAccountId": 75,
"bankName": "BARCLAYS BANK UK PLC",
"balanceAmount": 1500000.00,
"pendingAmount": 0.0,
"minBalance": 450,
"currency": "GBP",
"iban": "GB33BUKB20201555555555"
}
]
}
Returns all accounts owned and consented to by the end user (account owner). Account balance will only be returned if the end user has consented to the application accessing the account's information.
HTTP Request
GET https://api.brucbond.com/api/v1/accounts/all
Header Parameters
Parameter | Description |
---|---|
app-key | The application's client ID provided when registering your application. |
app-secret | The application's client secret provided when registering your application. |
Authorization | Authorization token granted during the authorization process. |
Get Single Account
curl -X GET "https://api.brucbond.com/api/v1/accounts/45/balances?currencyId=EUR"
-H "Content-Type: application/json"
-H "Authorization: Bearer <ACCESS_TOKEN>"
-H "app-key: <APPLICATION_KEY>"
-H "app-secret: APPLICATION_SECRET"
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true,
"result":
[
{
"balanceAmount": 100155.11,
"pendingAmount": 32654.15,
"minBalance": 500,
"currency": "EUR",
"iban": "321532543675"
}
]
}
Returns the balance of each currency held in the client's bank account, based on the provided {accountId}
and {currencyId}
.
HTTP Request
GET https://api.brucbond.com/api/v1/accounts/{accountId}/balances?currencyId={CurrencyId}
Header Parameters
Parameter | Description |
---|---|
app-key | The application's client ID provided when registering your application. |
app-secret | The application's client secret provided when registering your application. |
Authorization | Authorization token granted during the authorization process. |
Query Parameters
Parameter | Description |
---|---|
currencyId | Declares the currency of the required bank account. See Currency_code validator. |
Get Account Transactions
curl -X GET "https://api.brucbond.com/api/v1/accounts/{accountId}/transactions/range?currencyId=EUR&startdate=20190601Z&enddate=20190615Z"
-H "Content-Type: application/json"
-H "Authorization: Bearer <ACCESS_TOKEN>"
-H "app-key: <APPLICATION_KEY>"
-H "app-secret: <APPLICATION_SECRET>"
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true,
"result":
[
{
"id": 512461,
"source": 3,
"company": "Reimer GmbH",
"counterparty":
{
"name": "Appel Wilhelm KG",
"country":
{
"name": "Germany",
"dialCode": "+49",
"code": "DE"
},
"address": "Luise-Brückner-Platz 89b00129 Ratingen"
},
"bankDetails":
{
"name": "Commerzbank",
"country":
{
"name": "Germany",
"dialCode": "+49",
"code": "DE"
},
"address": "Köln 50447",
"bic": "COBADEFF"
},
"intermediaryBank": "",
"accountNumber": "DE89370400440532013000",
"routingNumber": "",
"reason": "Salary payments Jun 19",
"createDate": "2019-06-11T16:58:29.57",
"lastModifyDate": "2019-06-13T11:30:36.403",
"amount": 130000,
"currency": "EUR",
"status": 1,
"isDebit": true,
"isUrgent": true
},
{
"id": 512789,
"source": 3,
"company": "Lang and Sons",
"counterparty":
{
"name": "Hamill, Zboncak and Weimann",
"country":
{
"name": "United Kingdom",
"dialCode": "+44",
"code": "UK"
},
"address": "38 Jayden DriveRoseburghBB4 5TZ"
},
"bankDetails":
{
"name": "BARCLAYS BANK UK PLC",
"country":
{
"name": "United Kingdom",
"dialCode": "+44",
"code": "UK"
},
"address": "Leicester LE87 2BB",
"bic": "BUKBGB22"
},
"intermediaryBank": "",
"accountNumber": "GB33BUKB20201555555555",
"routingNumber": "",
"reason": "Invoice ref num. 43256723",
"createDate": "2019-06-11T16:58:29.57",
"lastModifyDate": "2019-06-13T11:30:36.403",
"amount": 5000.00,
"currency": "EUR",
"status": 1,
"parentId": null,
"isDebit": true,
"isUrgent": false
}
]
}
status
andsource
values can be found in Enums section
Returns transactions information for a given account, based on the provided {accountId}
.
The operation limits the transactions to the date range set by the startDate
and endDate
parameters.
Transactions will only include one of the creditor and debtor properties, depending on the type of the transaction. The irrelevant field will be null.
HTTP Request
GET https://api.brucbond.com/api/v1/accounts/{accountId}/transactions/range?currencyId={CurrencyId}&startDate=yyMMddZ&endDate=yyMMddZ
Header Parameters
Parameter | Description |
---|---|
app-key | The application's client ID provided when registering your application. |
app-secret | The application's client secret provided when registering your application. |
Authorization | Authorization token granted during the authorization process. |
Query Parameters
Parameter | Description |
---|---|
currencyId | Declares the currency of the required bank account. See Currency_code validator. |
startDate | The beginning of the date-range for displaying transactions. (e.g: 190915Z = Sep 15, 2019 ) |
endDate | The end of the date-range for displaying transactions. (e.g: 190915Z = Sep 15, 2019 ) |
Payment Initiation (PIS)
Service propositions enabled by customers (PSUs) consenting to Payment Initiation Service Providers (PISPs) initiating payments from their payment accounts.
Payment Initiation Request
curl -X POST "https://api.brucbond.com/api/v1/payment"
-H "Content-Type: application/json"
-H "Authorization: Bearer <ACCESS_TOKEN>"
-H "app-key: <APPLICATION_KEY>"
-H "app-secret: <APPLICATION_SECRET>"
-d '{
"counterparty": {
"name": "Weber, Gulgowski and Turner",
"country": {
"code": "DE"
},
"address": "Kareemville, MS 67792"
},
"bankDetails": {
"name": "Commerzbank",
"country": {
"code": "DE"
},
"address": "Köln 50447",
"bic": "COBADEFF"
},
"intermediaryBank": null,
"accountNumber": "DE89370400440532013000",
"amount": 12500,
"currency": "EUR",
"routingNumber": "124566457889",
"reason": "INVOICE 04 2018 - Directors Fees",
"isUrgent": false
}'
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true,
"result": {
"transactionId": "cd2c8a30-055d-4628-b5f0-30265f4f2209"
}
This operation will create a payment initiation request at Brüc + Bond as a resource with all data relevant for the corresponding payment.
HTTP Request
POST https://api.brucbond.com/api/v1/payment
Header Parameters
Parameter | Description |
---|---|
app-key | The application's client ID provided when registering your application. |
app-secret | The application's client secret provided when registering your application. |
Authorization | Authorization token granted during the authorization process. |
Body Parameters
Level | Parameter | Type | Description |
---|---|---|---|
* | counterparty | Object |
Counter-party details. |
** | name | String (M) |
Counter-party full name (first name and surname in case of individual person or company name of legal body). See String Validator. |
** | countryCode | String (M) |
ISO 3166-1 alpha-2 country code of counter-party address. See Country_code validator. |
** | address | String (M) |
Counter-party’s address, usually consists of street name, building number, ZIP code, city name, country name. See String Validator. |
* | bankDetails | Object |
Counter-party's bank details. |
** | name | String (C) |
Name of counter-party's bank. Optional if "accountNumber" is a valid IBAN. See String Validator. |
** | countryCode | String (C) |
ISO 3166-1 alpha-2 country code of counter-party's bank address. Optional if "accountNumber" is a valid IBAN. See Country_code validator. |
** | address | String (C) |
Counter-party’s bank address, usually consists of street name, building number, ZIP code, city name, country name. Optional if "accountNumber" is a valid IBAN. See String Validator. |
** | bic | String (C) |
BIC code of counter-party's bank. Optional if "accountNumber" is a valid IBAN. See BIC Validator. |
* | intermediaryBank | String (O) |
An intermediary bank is any bank between the issuing bank and the beneficiary bank. Adding an intermediary bank to a beneficiary describes the precise path a payment is to follow to reach a beneficiary bank account. There is no intermediary bank for local or SEPA payments. See String Validator. |
* | accountNumber | String (M) |
Account number (IBAN / BBAN) of the counter-party. See Alphanumeric validator. |
* | amount | Decimal (M) |
The amount given with fractional digits, where fractions must be compliant to the currency definition. See Positive_decimal validator. |
* | currency | String (M) |
Transaction amount ISO 4217 currency code. See Currency_code validator. |
* | routingNumber | String (O) |
Bank clearing number (ABA, FW, CNAPS, etc.), used usually when sending money to North America. See Alphanumeric validator. |
* | reason | String (M) |
Purpose of the payment (usually includes a reference to a provided service or goods). See String Validator. |
* | isUrgent | Boolean (M) |
A transaction marked as urgent will be sent express at the bank, ahead of the next scheduled processing time. Possible values: true , false . |
Payment Confirmation
curl -X POST "https://api.brucbond.com/api/v1/payment/confirm"
-H "Content-Type: application/json"
-H "Authorization: Bearer <ACCESS_TOKEN>"
-H "app-key: <APPLICATION_KEY>"
-H "app-secret: <APPLICATION_SECRET>"
-d '{
"transactionId": "cd2c8a30-055d-4628-b5f0-30265f4f2209",
"code": "882968"
}'
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true
}
This endpoint confirms the payment initiation is not fradulent, through a one-time password sent via SMS to the client.
HTTP Request
POST https://api.brucbond.com/api/v1/payment/confirm
Header Parameters
Parameter | Description |
---|---|
app-key | The application's client ID provided when registering your application. |
app-secret | The application's client secret provided when registering your application. |
Authorization | Authorization token granted during the authorization process. |
Body Parameters
Level | Parameter | Type | Description |
---|---|---|---|
* | transactionId | String (M) |
Unique identifying number of the transaction from Payment initiation request. |
* | code | String (M) |
One-time password which was sent to the client via SMS. |
Confirmation Availability of Funds (CBPII)
Funds Confirmation
curl "https://api.brucbond.com/api/v1/accounts/{accountId}/check-funds?currencyId=EUR&amount=322.32"
-H "Content-Type: application/json"
-H "Authorization: Bearer <ACCESS_TOKEN>"
-H "app-key: <APPLICATION_KEY>"
-H "app-secret: <APPLICATION_SECRET>"
The above command returns JSON structured like this:
{
"version": "4.0.0",
"statusCode": 200,
"success": true,
"result": false
}
A Card Based Payment Instrument Issuer is a payment services provider that issues card-based payment instruments,
that can be used to initiate a payment transaction from a payment account (based on the provided {accountId}
) held with another payment service provider.
Service propositions enabled by customers (PSUs) giving their consent to a CBPII to submit Confirmation of Funds (CoF) requests to an ASPSP.
HTTP Request
GET https://api.brucbond.com/api/v1/accounts/{AccountId}/check-funds?currencyId={CurrencyId}&amount={Amount}
Header Parameters
Parameter | Description |
---|---|
app-key | The application's client ID provided when registering your application. |
app-secret | The application's client secret provided when registering your application. |
Authorization | Authorization token granted during the authorization process. |
Query Parameters
Parameter | Description |
---|---|
currencyId | Declares the currency of the required bank account. See Currency_code validator. |
amount | The amount of the declared currency that needs to be available to credit. See Positive_decimal validator. |
Enums
Transaction Status Enums
Enum | Meaning |
---|---|
1 | Pending |
2 | Settled / Completed |
3 | Rejected |
5 | Waiting to be signed. |
Transaction source Enums
Enum | Meaning |
---|---|
1 | FX sell |
2 | Fx buy |
3 | Outgoing (debit) |
4 | Incoming (credit) |
5 | Fee |
6 | Correction (credit) |
7 | Correction (debit) |
10 | Refund (credit) |
11 | Refund (fee, credit) |
12,13,14 | Fix fee (monthly, additional user, setup) |
Validations
Validator | Meaning |
---|---|
String | Up to 100 characters. allows only alphanumeric characters (A-Z, a-z, 0-9) whitespace and some of the special characters (. , ( )_ -). |
Alphanumeric | Up to 100 characters. allows only alphanumeric characters (A-Z, a-z, 0-9). |
Country_code | 2 UPPERCASE characters. ISO 3166-1 alpha-2 country code. |
Positive_decimal | Amount (D:2) Positive greater than zero decimal number. |
BIC | ISO 9362 BIC (Business Identifier Code). BICs can be 8 or 11 characters in length and contain only UPPERCASE letters or digits. |
Currency_code | ISO 4217 currency code; Length: 3 UPPERCASE letters. |
Account_number | An ISO 13616:2007 valid IBAN OR valid account number / BBAN (alphanumeric (A-Z, a-z, 0-9). |
Errors
Error Code | Meaning |
---|---|
400 | Bad Request - Your request is invalid. |
401 | Unauthorized - Your API key is wrong. |
403 | Forbidden - The data requested is hidden for administrators only. |
404 | Not Found - The specified data could not be found. |
405 | Method Not Allowed - You tried to access data with an invalid method. |
406 | Not Acceptable - You requested a format that isn't json. |
410 | Gone - The data requested has been removed from our servers. |
429 | Too Many Requests. |
500 | Internal Server Error - We had a problem with our server. Try again later. |
503 | Service Unavailable - We're temporarily offline for maintenance. Please try again later. |